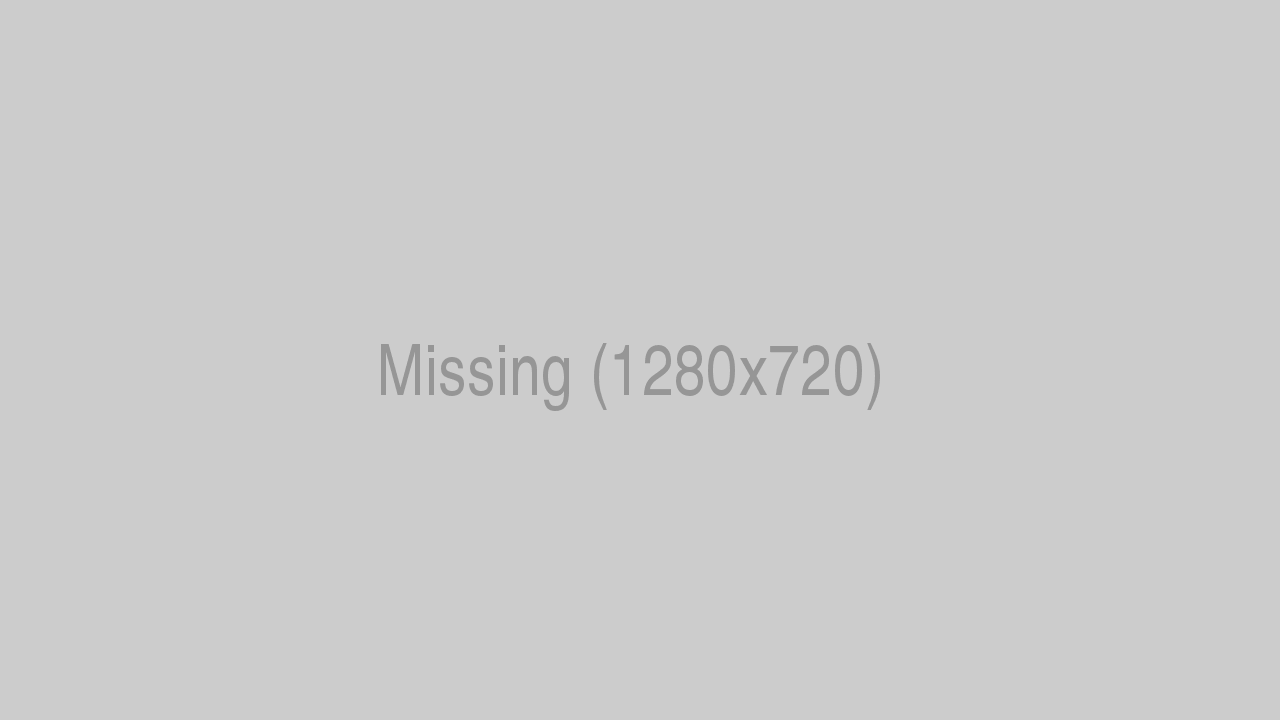
To access the full video please subscribe to FLLCasts.com
- #1550
- 06 Mar 2020
Example solution to the task "Display "Hello World" text on the brick screen for 3 seconds. Experiment with the coordinates until the text appears approximately in the middle of the screen"
# Create your objects here. ev3 = EV3Brick() # Write your program here. ev3.screen.draw_text(40, 50, "Hello World") wait(3000)
Example solution to the task "Create a numeric variable named "hours" equal to 2 and use the ev3.screen.draw_text() command to display it on the screen. You don't need to put variables in quotes."
# Create your objects here. ev3 = EV3Brick() hours = 2 # Write your program here. ev3.screen.draw_text(40, 50, hours) wait(3000)
Example solution to the task "Program the "hours" variable to be a randomly selected number between 1 and 12. Test your program and tell us what number did you saw on the display."
import random # Create your objects here. ev3 = EV3Brick() hours = random.randint(1, 12) # Write your program here. ev3.screen.draw_text(40, 50, hours) wait(3000)
Example solution to the task "Create another variable and name it "minutes" equal to a random number between 1 and 60. Show it on the screen after the "hours" variable. Test your program and tell us what number did you saw on the display."
import random # Create your objects here. ev3 = EV3Brick() hours = random.randint(1, 12) minutes = random.randint(1, 60) # Write your program here. ev3.screen.draw_text(40, 50, hours) ev3.screen.draw_text(40, 70, minutes) wait(3000)
Example solution to the task "How many degrees does the short arrow of the clock have to rotate to show that an hour has passed? (360/12)"
360 / 12 = 30
Example solution to the task "How many degrees does the long arrow of the clock have to rotate to show that one minute has passed? (360/60)"
360 / 60 = 6
Example solution to the task "Create a variable named "hour_degrees" equal to the randomly selected number of hours in the variable "hours" multiplied by (360/12)"
import random # Create your objects here. ev3 = EV3Brick() hours = random.randint(1, 12) minutes = random.randint(1, 60) hour_degrees = hours * (360/12)
Example solution to the task "Create another variable named "minutes_degrees" equal to the randomly selected number of minutes in the variable "minutes" multiplied by (360/60)."
import random # Create your objects here. ev3 = EV3Brick() hours = random.randint(1, 12) minutes = random.randint(1, 60) hour_degrees = hours * (360/12) minute_degrees = minutes * (360/60)
Example solution of the task "Program the robot's short arrow to move a number of degrees equal to "hour_degrees" with a speed of 360."
import random # Create your objects here. ev3 = EV3Brick() hours = random.randint(1, 12) minutes = random.randint(1, 60) hour_degrees = hours * (360/12) minute_degrees = minutes * (360/60) # Write your program here. Motor(Port.B).run_angle(360, hour_degrees, Stop.COAST)
Example solution to the task "Using a keyboard shortcut, copy the line with the run_angle () command and paste it underneath it. Change the copied line so that the long arrow (motor C) of the robot moves a number of degrees equal to "minute_degrees" with a speed of 360."
import random # Create your objects here. ev3 = EV3Brick() hours = random.randint(1, 12) minutes = random.randint(1, 60) hour_degrees = hours * (360/12) minute_degrees = minutes * (360/60) # Write your program here. Motor(Port.B).run_angle(360, hour_degrees, Stop.COAST, False) Motor(Port.C).run_angle(360, minute_degrees, Stop.COAST)
Example solution to the task "Extract your motors in variables with the names "hours_motor" and "minutes_motor"."
import random # Create your objects here. ev3 = EV3Brick() hours_motor = Motor(Port.B) minutes_motor = Motor(Port.C) hours = random.randint(1, 12) minutes = random.randint(1, 60) hour_degrees = hours * (360/12) minute_degrees = minutes * (360/60) # Write your program here. hours_motor.run_angle(360, hour_degrees, Stop.COAST, False) minutes_motor.run_angle(360, minute_degrees, Stop.COAST)
Example solution to the task "Program the robot to wait 5 seconds after moving its arrows and then turn them back."
import random # Create your objects here. ev3 = EV3Brick() hours_motor = Motor(Port.B) minutes_motor = Motor(Port.C) hours = random.randint(1, 12) minutes = random.randint(1, 60) hour_degrees = hours * (360/12) minute_degrees = minutes * (360/60) # Write your program here. hours_motor.run_angle(360, hour_degrees, Stop.COAST, False) minutes_motor.run_angle(360, minute_degrees, Stop.COAST) wait(5000) hours_motor.run_angle(-360, hour_degrees, Stop.COAST, False) minutes_motor.run_angle(-360, minute_degrees, Stop.COAST)
Example solution to the task "Program the hours and minutes not to appear on the screen before the clock hands are in their correct positions for 5 seconds."
import random # Create your objects here. ev3 = EV3Brick() hours_motor = Motor(Port.B) minutes_motor = Motor(Port.C) hours = random.randint(1, 12) minutes = random.randint(1, 60) hour_degrees = hours * (360/12) minute_degrees = minutes * (360/60) # Write your program here. hours_motor.run_angle(360, hour_degrees, Stop.COAST, False) minutes_motor.run_angle(360, minute_degrees, Stop.COAST) wait(5000) ev3.screen.draw_text(40, 50, hours) ev3.screen.draw_text(40, 70, minutes) wait(3000) hours_motor.run_angle(-360, hour_degrees, Stop.COAST, False) minutes_motor.run_angle(-360, minute_degrees, Stop.COAST)
Courses and lessons with this Tutorial
This Tutorial is used in the following courses and lessons
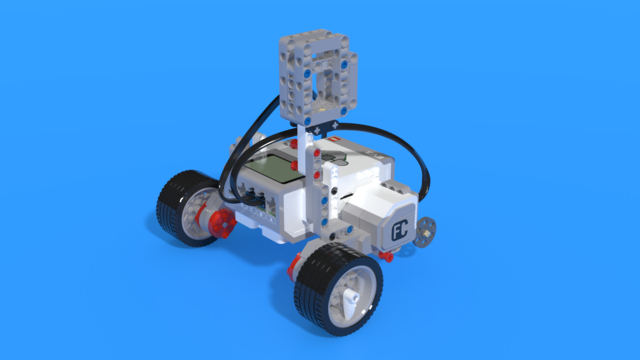
Python with LEGO Mindstorms EV3 - Level 1
The course introduces students to the programming language Python. We use LEGO Mindstorms EV3 Robots. Python is a popular programming language. It could be used for introducing students to programming, for academic studies, for developing machine learning algorithms and as a general-purpose language.
During the course, students learn how to read and how to develop Python programs. They use an Integrated Development Environment called Visual Studio Code. Robots are programmed to perform interesting and funny tasks like "bringing you water". The level ends with competition on a playing field with boxes.
- 74
- 28:18
- 114
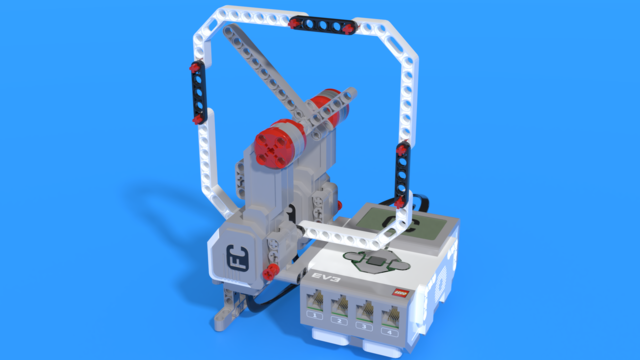
Lesson 5 - Educational toy: Learn to read the clock
Returning feedback to students is important. We try to return feedback constantly in the classes. You should also try to return feedback in a structured way - in a form of a digit. Today you will have to grade your students following this article.
- 7
- 10
- 16
- 3d_rotation 1