Solutions to the programming tasks in this lesson.
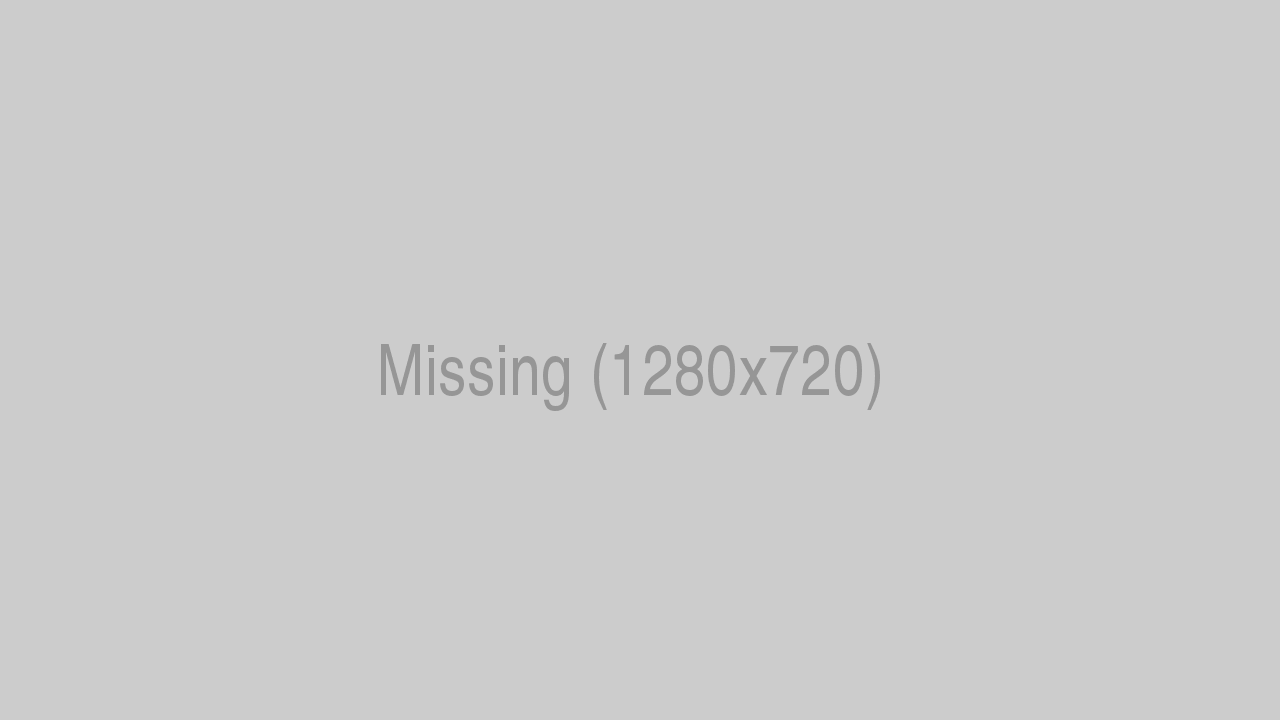
To access the full video please subscribe to FLLCasts.com
- #1620
- 12 Jun 2020
Example solution to the tasks in the section "Gesture detection from left to right":
# Create your objects here. ev3 = EV3Brick() left_touch = TouchSensor(Port.S1) right_touch = TouchSensor(Port.S2) left_motor = Motor(Port.B) right_motor = Motor(Port.C) # Write your program here. ev3.speaker.beep() while not left_touch.pressed(): pass while left_touch.pressed(): pass wait(200) if right_touch.pressed(): left_motor.run(1000) right_motor.run(1000) wait(2000)
Example solution to the task "Change your program so that the robot detects gestures from right to left.":
# Create your objects here. ev3 = EV3Brick() left_touch = TouchSensor(Port.S1) right_touch = TouchSensor(Port.S2) left_motor = Motor(Port.B) right_motor = Motor(Port.C) # Write your program here. ev3.speaker.beep() while not right_touch.pressed(): pass while right_touch.pressed(): pass wait(200) if left_touch.pressed(): left_motor.run(1000) right_motor.run(1000) wait(2000)
Example solution to the tasks in the section "Dynamic detection of gestures from left to right":
# Create your objects here. ev3 = EV3Brick() left_touch = TouchSensor(Port.S1) right_touch = TouchSensor(Port.S2) left_motor = Motor(Port.B) # Write your program here. right_motor = Motor(Port.C) # Write your program here. ev3.speaker.beep() while True: if left_touch.pressed(): while left_touch.pressed(): pass wait(200) if right_touch.pressed(): left_motor.run_angle(1000, 360, Stop.HOLD, False) right_motor.run_angle(1000, 360)
Example solution to the task "Program another check inside the loop, that lets the robot check right-to-left gestures.":
# Create your objects here. ev3 = EV3Brick() left_touch = TouchSensor(Port.S1) right_touch = TouchSensor(Port.S2) left_motor = Motor(Port.B) right_motor = Motor(Port.C) # Write your program here. ev3.speaker.beep() while True: if left_touch.pressed(): while left_touch.pressed(): pass wait(200) if right_touch.pressed(): left_motor.run_angle(1000, 360, Stop.HOLD, False) right_motor.run_angle(1000, 360) if right_touch.pressed(): while right_touch.pressed(): pass wait(200) if left_touch.pressed(): left_motor.run_angle(1000, -360, Stop.HOLD, False) right_motor.run_angle(1000, -360)
Courses and lessons with this Tutorial
This Tutorial is used in the following courses and lessons
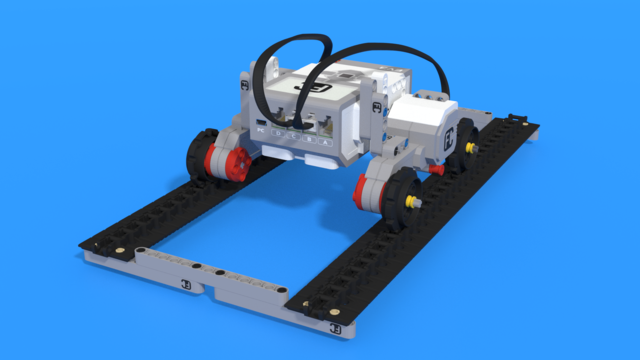
Python with LEGO Mindstorms EV3 - Level 2
In the second level of Python for EV3 robots, students learn in-depth the touch sensor. The sensor is used as an input device for manual control of machines, as well as a sensor for autonomous robots. In a pair of lessons, students build a control panel for the grabber and the movement of a crane. Programming wise, students learn how to fork code with "if-else" constructions, how to create conditional and forever loops with "while" and how to negate conditions with "not" operator. In the end of the lesson, robots can detect obstacles and avoid them, so that they traverse a simple labyrinth.
- 39
- 19:58
- 93
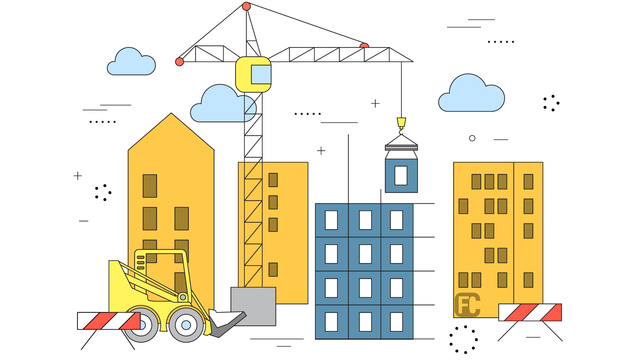
Lesson 3 - Crane movement control panel
Introduction
Today, we will continue building upon the control console from the previous lesson by creating a robot that is controlled using gestures.
Some gesture sensors work by briefly activating nearby sensors. You will be using the touch sensor to simulate this process and create your own gesture sensor.
- 3
- 4
- 6
- 3d_rotation 2